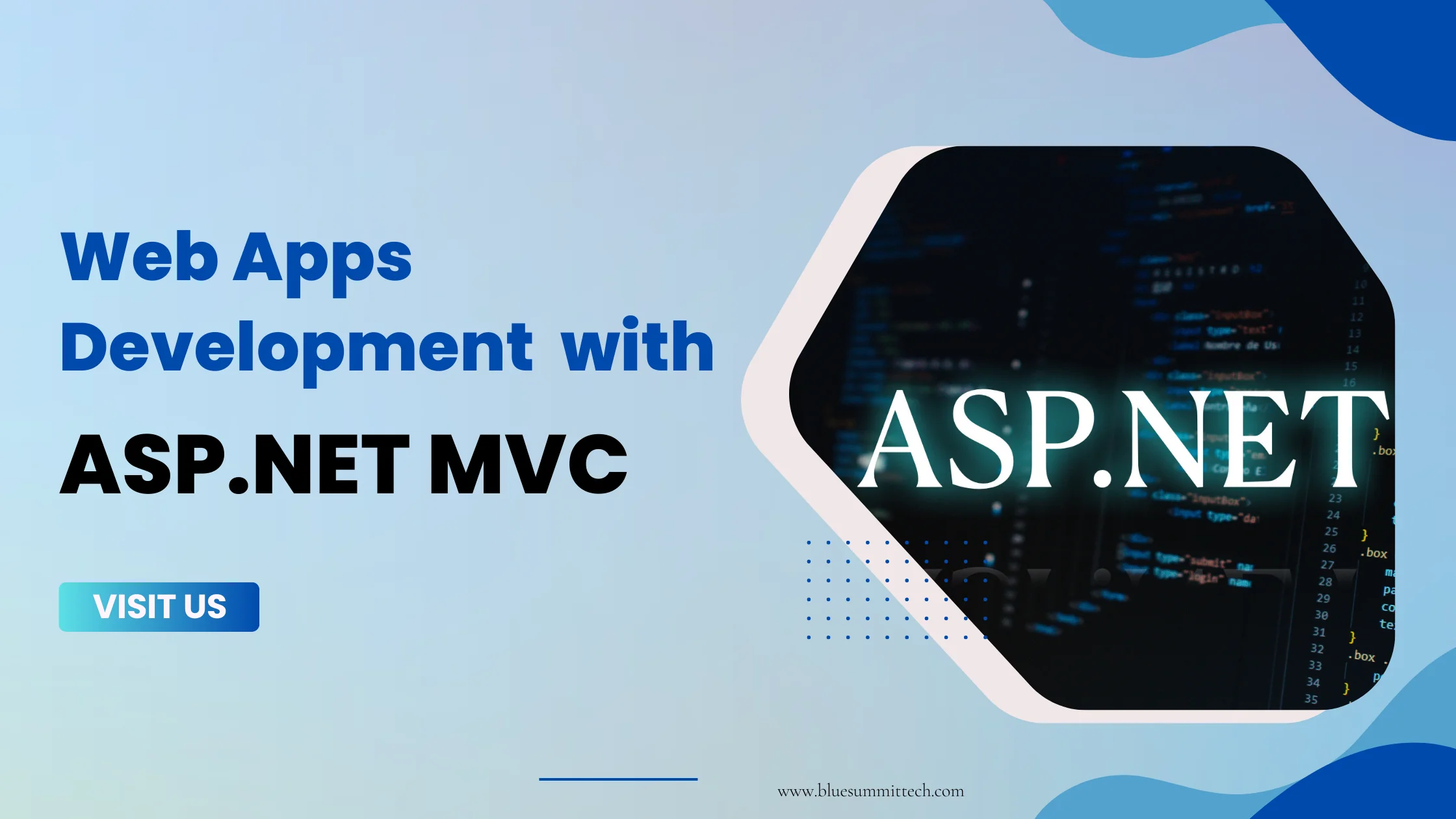
Posted on Wednesday, Jun 07th, 2023
Understanding ASP.NET MVC: An Overview
Spread the love
Introduction to MVC architectural pattern
In the realm of web application development, the Model-View-Controller (MVC) architectural pattern has emerged as a powerful paradigm for building robust and maintainable applications. ASP.NET MVC, a framework developed by Microsoft, embraces this pattern and provides developers with a structured approach to creating dynamic and scalable web applications.At its core, the MVC pattern separates an application into three distinct components: the Model, the View, and the Controller. Each component has a specific responsibility, enabling a clear separation of concerns and promoting code organization and reusability.
Key components: Model, View, and Controller
Model
The Model represents the application's data and business logic. It encapsulates the state of the application and provides the necessary operations to manipulate and retrieve data. In ASP.NET MVC, the Model is often implemented using classes that interact with a database, XML files, or other data sources.View
The View is responsible for presenting the data to the user in a visually appealing and interactive manner. It focuses on the user interface and rendering the model data. In ASP.NET MVC, views are typically created using the Razor view engine, which combines HTML markup with server-side code to generate dynamic content.Controller
The Controller acts as an intermediary between the Model and the View. It handles user requests, processes user input, and determines the appropriate response. The Controller interacts with the Model to fetch or update data and then passes that data to the View for rendering. It plays a crucial role in coordinating the application flow and enforcing business rules.ASP.NET MVC embraces the principles of the MVC pattern and provides a framework that simplifies the development process. It includes various features such as routing, data binding, and automatic generation of HTML forms, which streamline the implementation of MVC-based applications.
By adopting the MVC pattern, developers can achieve several benefits. The separation of concerns allows for easier maintenance and testing of individual components. Changes to the user interface can be made independently of the underlying data and business logic, promoting flexibility and code reusability. Additionally, the structured nature of MVC facilitates collaboration among developers working on different components of the application.
Advantages of ASP.NET MVC
There are many advantages of ASP.NET MVC usage for web development over other frameworks, including:1. Scalability and Ease of Use
It enables developers to build dynamic, robust, and scalable web applications. It efficiently handles requests at runtime by monitoring and managing processes, replacing any inactive ones with new instances. This scalability ensures optimal performance even under heavy traffic. Additionally, ASP.NET simplifies common tasks such as form submission, client authentication, site configuration, and deployment, making it user-friendly for developers.2. Enhanced Security
Security is a top priority in application development, and it excels in this aspect. With built-in Windows authentication and per-application configuration, ASP.NET provides a secure framework for building applications. It ensures that sensitive data is protected, and robust authentication mechanisms are implemented.3. Developer-Friendly Features
It offers a range of features that enhance developer productivity. With a rich toolbox and designer in the Visual Studio integrated development environment (IDE), developers can efficiently create applications with minimal coding. The availability of features like automatic deployment, WYSIWYG (What You See Is What You Get) editing, and drag-and-drop server controls significantly streamline the development process.4. Maintainability
It promotes code maintainability and reusability. The framework separates application logic into distinct layers, such as models, views, and controllers, facilitating a clean and modular code structure. This separation of concerns makes it easier to update and maintain different parts of the application individually.5. Cross-Platform Compatibility
ASP.NET Core, the latest version of ASP.NET, is cross-platform compatible. It can be used to develop applications that can run on Windows, Linux, and macOS. This flexibility allows developers to target a broader range of platforms and reach a larger audience.6. Performance Optimization
This includes features like caching, just-in-time compilation, and dynamic output caching that contribute to improved performance. These optimizations reduce server round-trips and enhance response times, ensuring a smooth user experience.7. Integrated Development Environment (IDE)
It integrates seamlessly with Visual Studio, a robust IDE with powerful debugging, testing, and profiling tools. Visual Studio provides a comprehensive development environment that enhances productivity and makes application development more efficient.Prominent Features of ASP.NET MVC
1. Separation of Concerns
It follows the MVC architectural pattern, which promotes a clear separation of concerns. The model represents the data and business logic, the view handles the presentation and user interface, and the controller manages the flow of the application. This separation allows for better code organization, modularity, and maintainability.2. Routing
This includes a powerful routing system that enables developers to define custom URL patterns for their application. The routing engine maps incoming URLs to specific controller actions, allowing for clean and search engine-friendly URLs. This feature enhances the overall user experience and improves the application's accessibility.3. Testability
This is designed with testability in mind. The separation of concerns makes it easier to write unit tests for individual components of the application, such as controllers and models. The framework provides built-in support for test-driven development (TDD), making it effortless to write automated tests and ensure the reliability and correctness of the application.4. Extensibility
It offers a highly extensible architecture, allowing developers to customize and extend its functionality to meet specific requirements. Developers can create custom model binders, filters, view engines, and more, enabling them to tailor the framework to their needs. This extensibility empowers developers to build highly flexible and adaptable web applications.5. Razor View Engine
ASP.NET MVC introduced the Razor view engine, which provides a concise and expressive syntax for creating dynamic and readable views. Razor combines HTML markup with server-side code seamlessly, making it easier to write and maintain views. It offers features like layouts, partial views, and HTML helpers that streamline the view development process.6. Integrated Security
It integrates with the ASP.NET security infrastructure, which includes features such as authentication, authorization, and role-based access control. Developers can leverage these built-in security mechanisms to secure their applications and protect sensitive data.7. Client-Side Development
It allows developers to incorporate client-side technologies such as HTML5, CSS, and JavaScript seamlessly. It provides support for popular JavaScript libraries and frameworks like jQuery and AngularJS, enabling developers to build rich and interactive user interfaces.8. Support for RESTful APIs
It is well-suited for building RESTful APIs. It provides features like attribute routing, content negotiation, and HTTP verb-specific action methods, making it easier to expose and consume web APIs. This feature enables developers to create scalable and interoperable web services.Tools and techniques
1. Visual Studio
Visual Studio is the primary Integrated Development Environment (IDE) for ASP.NET MVC development. It provides a comprehensive set of tools, including project templates, code editors, debugging capabilities, and built-in integration with source control systems.2. ASP.NET MVC Template
The ASP.NET MVC template is a project template provided by Visual Studio that sets up the basic structure and configuration for an MVC application. It includes essential files, folders, and configuration settings to get started with development quickly.3. Razor View Engine
Razor is the default view engine in ASP.NET MVC. It allows developers to combine HTML markup with server-side code seamlessly, providing a concise and expressive syntax for creating dynamic views. Razor simplifies the development and maintenance of views and supports features like layouts, partial views, and HTML helpers.4. Entity Framework (EF)
Entity Framework is an Object-Relational Mapping (ORM) framework that simplifies database access and management in ASP.NET MVC applications. It enables developers to work with databases using object-oriented techniques, providing features like automatic data modeling, query generation, and database migrations.5. Bundling and Minification
ASP.NET MVC includes built-in support for bundling and minification of static assets like CSS and JavaScript files. Bundling combines multiple files into a single bundle, reducing the number of HTTP requests, while minification compresses and optimizes the file size. These techniques improve page load times and overall performance.6. Routing
ASP.NET MVC's routing system maps incoming URLs to specific controller actions. It allows developers to define custom URL patterns and handle different types of HTTP requests (GET, POST, etc.) easily. Routing enhances the application's usability, search engine optimization, and supports the creation of clean and user-friendly URLs.7. Action Filters
Action filters provide a mechanism to inject custom logic before or after an action method executes in ASP.NET MVC. They enable developers to implement cross-cutting concerns such as authorization, logging, caching, and exception handling. Action filters help keep the code modular, reusable, and maintainable.8. Dependency Injection (DI)
It supports dependency injection, which allows for the loose coupling of application components. Developers can utilize a DI container like Autofac, Ninject, or Microsoft.Extensions.DependencyInjection to manage dependencies and improve testability, modularity, and extensibility.9. Unit Testing Frameworks
Various unit testing frameworks, such as NUnit, MSTest, and xUnit, can be used in ASP.NET MVC to write automated tests for controllers, models, and other components. These frameworks facilitate the creation and execution of unit tests, ensuring the correctness and reliability of the application.10. Version Control Systems
Version control systems like Git or Team Foundation Version Control (TFVC) are commonly used in the development to track and manage changes to source code. They enable collaboration, code branching, merging, and provide a history of changes, ensuring code integrity and facilitating teamwork.Best practices of ASP.NET MVC Development
When developing web applications using ASP.NET MVC, following best practices can greatly improve code quality, maintainability, and overall application performance. Here are some essential best practices to consider:i. Separation of Concerns
Follow the MVC architectural pattern strictly, ensuring a clear separation of concerns between the Model, View, and Controller. Each component should have a specific responsibility, keeping the codebase organized and making it easier to maintain and test.ii. Maintain Clean and Readable Code
Write clean, readable, and self-explanatory code. Use meaningful variable and method names, adhere to consistent coding conventions, and properly comment your code to enhance its readability and maintainability.iii. Keep Controllers Thin
Controllers should primarily handle user input, application flow, and interaction with the Model and View. Avoid placing complex business logic or data access code in controllers. Instead, move such functionality to appropriate service or repository classes, promoting separation of concerns and easier unit testing.iv. Leverage Strongly-Typed Views
Utilize strongly-typed views with view models to pass data from the controller to the view. This approach enhances compile-time safety, improves code clarity, and helps prevent runtime errors caused by passing incorrect data to the view.v. Utilize Validation
Implement validation at both the client-side and server-side to ensure data integrity and security. Use validation attributes provided by the .NET framework or create custom validation logic as needed. Validate user input and model data in controllers before processing or persisting it.vi. Implement Security Measures
Implement proper authentication and authorization mechanisms to protect your application. Utilize ASP.NET Identity for user management and role-based access control. Apply appropriate security measures, such as input sanitization, to prevent common security vulnerabilities like SQL injection and cross-site scripting (XSS) attacks.vii. Implement Caching
Utilize caching techniques to optimize performance and reduce server load. Apply output caching for frequently accessed data or views. Leverage browser caching, HTTP caching, or data caching where applicable to minimize redundant requests and improve overall response times.viii. Implement Unit Testing
Write unit tests for critical components of your application, such as controllers and models. Utilize testing frameworks like NUnit or MSTest to automate the testing process. Writing tests ensures code correctness, facilitates easier debugging, and promotes code maintainability and refactoring.ix. Optimize Performance
Apply performance optimization techniques like bundling and minification of JavaScript and CSS files. Use asynchronous programming where appropriate to improve responsiveness. Optimize database queries and leverage caching to minimize round-trips to the server. Monitor and analyze performance using tools like profiling and performance testing.x. Regularly Update Dependencies
Keep your application and its dependencies up-to-date with the latest stable versions. Update the .NET framework, NuGet packages, and third-party libraries to leverage bug fixes, security patches, and performance improvements.Ending Note
ASP.NET MVC provides developers with a powerful and structured framework for building dynamic and scalable web applications. By embracing the Model-View-Controller architectural pattern, ASP.NET MVC promotes code organization, separation of concerns, and testability. With this, you can create applications that are maintainable, extensible, and performant.Begin your journey today by visiting our website to learn more about the ASP.NET MVC framework.
Blue Summit has collaborated with OdiTek Solutions, a frontline custom software development company. It is trusted for its high service quality and delivery consistency. Visit our partner's page to720day and get your business streamlined.
REFER TO OTHER RELEVANT CONTENTS
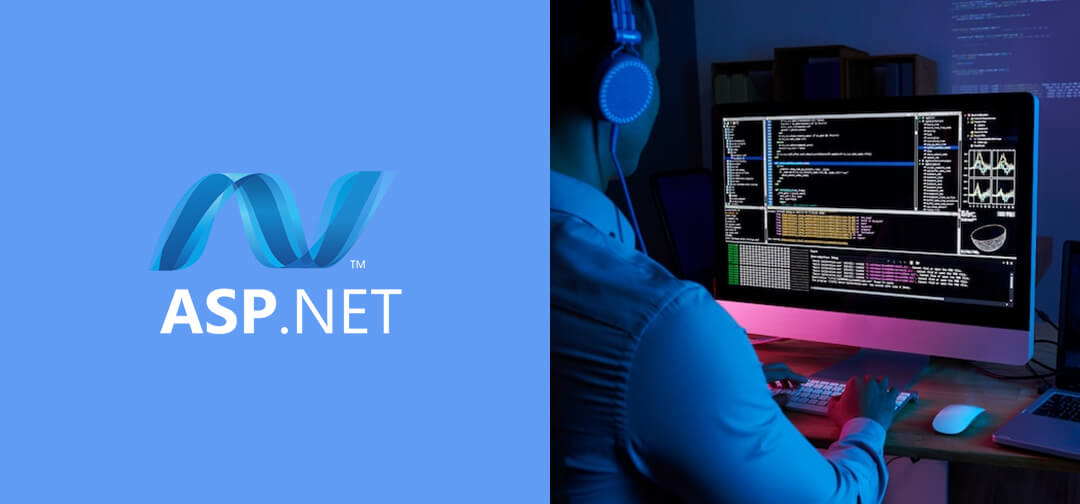
ASP.NET Development
Need a strong, adaptable, and reliable platform to carry your company into the next phase of growth and success? Microsoft created the open source ASP.NET (Active Server Pages.NET) framework primarily to aid programmers in creating dynamic, reactive, and responsive web pages and apps. When it...
read more